Basically I need a test vector with ideal sine wave, it should be 14 bit integer format and consists of 16 samples (a single sine period with maximum amplitude). I'm expecting symmetric AES17 sample representation which means that the max negative value is not used (for symmetry) and mean value should be 0.
Previously I was used test vector provided by another people, I don't know how it was generated, but it seems that this vector provides better sine performance than I can generate. Here it is:
0
3134
5792
7567
8191
7567
5792
3134
0
-3134
-5792
-7567
-8191
-7567
-5792
-3134
And it's spectrum:
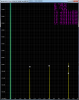
Now I'm trying to generate the same sine with code in the following way:
var bits = 14;
var halfRange = Math.Pow(2, bits-1);
for (var i = 0; i < 16; i++)
{
double sine = Math.Sin(2 * Math.PI * i / 16);
sample[i] = round( sine * (halfRange - 1D) );
}
sine * (halfRange - 1D) result:
0.0
3134.5599945024505
5791.91164469901
7567.49725079995
8191.0
7567.49725079995
5791.911644699011
3134.5599945024514
0.0000000000010030750644159092
-3134.5599945024496
-5791.91164469901
-7567.4972507999482
-8191.0
-7567.4972507999491
-5791.911644699012
-3134.5599945024555
and after round I get this:
0
3135
5792
7567
8191
7567
5792
3135
0
-3135
-5792
-7567
-8191
-7567
-5792
-3135
And it's spectrum:
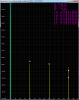
It seems that my sine has a little worse performance, it has -87.91 dB for 3'rd harmonic, while first sine has -95.83 dB for 3'rd harmonic.
Both test vectors almost the same, the only difference is 3135 instead of 3134. But I have no idea how to obtain 3134 value, because 3134.5599945 should be rounded to 3135. And if I replace round with floor (cut-off fraction part), I will get incorrect value for the third value, because 5791.91 will be rounded to 5791, but it should be 5792...
So there are two questions:
1) Is the first sine really has better performance? (maybe my spectrum calculation is just not so accurate)
2) How to calculate it with proper rounding?